Webpack for Web Development: How It Solves Challenges in 2025
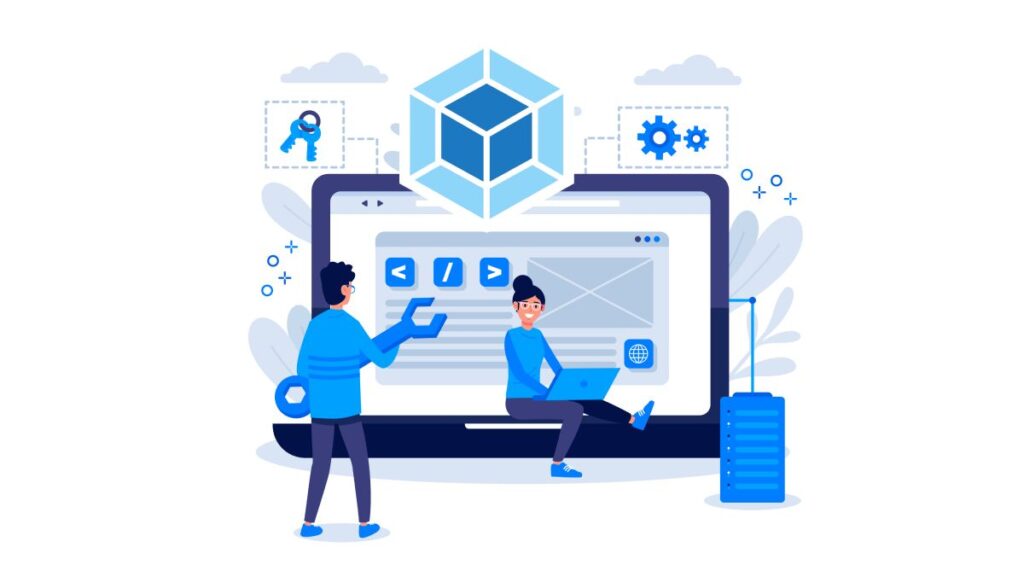
In the rapidly evolving world of web development, managing complex projects that involve multiple files, dependencies, and assets can quickly become overwhelming. This is where Webpack steps in as an indispensable tool.
Table of Contents
What is Webpack?
Webpack is a module bundler for modern JavaScript applications. Think of it as a powerful tool that takes all the code files and assets in your project (like JavaScript, CSS, and images) and bundles them into a single or a few files called “bundles.” These bundles are then ready to be used in your web application.
The key feature of Webpack is its ability to understand dependencies between files, ensuring everything is included and loaded in the right order.
Why Do We Need Webpack?
As web development has advanced, apps have become increasingly complex, often involving multiple JavaScript files, stylesheets, images, fonts, and other assets. Managing all of these manually is error-prone and inefficient.
Here’s why you need Webpack:
- Dependency Management: Imagine you have a project where file A depends on file B, which in turn depends on file C. Without Webpack, you would need to manually ensure that these files are loaded in the right order. Webpack automates this by understanding these dependencies and bundling everything together.
- Performance Optimization: Webpack can optimize your application for performance by reducing the size of files, minifying the code, and removing unnecessary parts (like unused functions). This makes your app load faster.
- Development Tools: Webpack offers features like Hot Module Replacement (HMR), which allows you to make changes in your code without refreshing the entire page, speeding up development significantly.
What Problems Does Webpack Solve?
Let’s look at the common challenges faced by web developers that Webpack effectively solves:
- Scattered Assets and Dependencies
In a typical web application, you have several assets such as JavaScript, CSS, images, and fonts that need to be linked correctly. Manually managing these can lead to missing files, incorrect order of loading, or bloated code. Webpack handles this by analyzing dependencies and ensuring everything is included properly. - Poor Performance in Production
When you’re ready to ship your application, performance is crucial. Large JavaScript files can slow down load times, leading to poor user experience. Webpack can minify and compress files, making them much smaller, while also splitting your code into smaller bundles that are loaded only when needed, a process called code splitting. - Redundant Code in Production
In large projects, there might be unused code that unnecessarily bloats the final output. Webpack includes a feature called tree shaking, which removes unused code to reduce the size of your final bundle. - Multiple Development Environments
Projects typically have different settings for development and production environments. For instance, in development, you want detailed error messages and easy debugging, while in production, you want optimized code. Webpack can be configured to handle both environments seamlessly.
Example: How Webpack Works
Let’s break down a simple example where Webpack is used to bundle a small project with JavaScript and CSS files.
- Project Structure:
/src
├── index.js
├── style.css
├── app.js
/dist
└── bundle.js (This will be generated by Webpack)
webpack.config.js
2. Installing Webpack:
First, install Webpack and Webpack CLI through npm:
npm install webpack webpack-cli --save-dev
3.Configuration:
In the webpack.config.js
file, you define how Webpack should bundle the files:
const path = require('path');
module.exports = {
entry: './src/index.js', // Entry point of your app
output: {
filename: 'bundle.js', // Name of the bundled file
path: path.resolve(__dirname, 'dist'), // Output directory
},
module: {
rules: [
{
test: /\.css$/, // Regex to match CSS files
use: ['style-loader', 'css-loader'], // Loaders to handle CSS
},
],
},
};
4. Running Webpack:
Once the config is set up, you can bundle your files by running:
npx webpack --config webpack.config.js
This will bundle index.js
and any of its dependencies into a single bundle.js
file in the /dist
folder, along with any required CSS.
Which Type of Websites Should Use Webpack?
Not every website needs Webpack. It shines in projects where complexity and scale are high. Here are some scenarios where Webpack is ideal:
- Single Page Applications (SPAs):
Web apps built with frameworks like React, Vue.js, or Angular often have complex JavaScript ecosystems with numerous components and dependencies. Webpack ensures that these dependencies are bundled efficiently. - Progressive Web Apps (PWAs):
PWAs rely on efficient asset management to load offline and provide a fast user experience. Webpack can manage caching strategies and asset bundling for PWAs. - Large, Content-heavy Websites:
If your website has a lot of images, fonts, and stylesheets, Webpack can optimize asset loading and ensure everything is compressed for faster load times. - JavaScript-heavy Custom Web Applications:
Any project where you use modern JavaScript features (ES6+) or TypeScript can benefit from Webpack’s module bundling and compatibility features.
Conclusion
In today’s world of fast, complex web applications, Webpack for Web Development is an essential tool for managing assets and dependencies. Whether you’re working on a small project or a large-scale app, Webpack for Web Development simplifies the process by bundling your files, optimizing performance, and providing features that improve both development and production workflows.
If you haven’t started using Webpack yet, now is the time to explore it and see how it can improve your development process and the performance of your web projects.
To enhance your coding skills and write cleaner, more maintainable JavaScript code, check out our article on 10 JavaScript Tips for Cleaner and More Maintainable Code.
FAQ
What is Webpack?
Webpack is a module bundler for modern JavaScript applications. It takes all the code files and assets in your project (like JavaScript, CSS, and images) and bundles them into one or a few files called “bundles,” which are then ready to be used in your web application.
Why do we need Webpack?
Webpack is essential for managing complex web applications that involve multiple files and assets. It automates dependency management, optimizes performance, and provides development tools that enhance the development experience.
What are the key features of Webpack?
Dependency Management: Automatically handles the loading order of files based on their dependencies.
Performance Optimization: Reduces file sizes, minifies code, and removes unnecessary parts to improve load times.
Development Tools: Offers features like Hot Module Replacement (HMR) for faster development.
What problems does Webpack solve?
Scattered Assets and Dependencies: Webpack ensures all assets are linked correctly and included in the final bundle.
Poor Performance in Production: It minifies and compresses files, improving load times.
Redundant Code in Production: Features like tree shaking remove unused code to reduce bundle size.
Multiple Development Environments: Webpack can be configured to handle different settings for development and production seamlessly.
Which types of websites should use Webpack?
Webpack is ideal for:
Single Page Applications (SPAs): Built with frameworks like React, Vue.js, or Angular.
Progressive Web Apps (PWAs): Require efficient asset management for offline loading.
Large, Content-heavy Websites: Optimize asset loading and compression.
JavaScript-heavy Custom Web Applications: Projects using modern JavaScript features or TypeScript.
How does Webpack improve the development process?
Webpack simplifies the development process by automating asset management, optimizing performance, and providing tools that enhance both development and production workflows. It allows developers to focus on writing code rather than managing dependencies and assets manually.
Is Webpack necessary for all web projects?
No, not every website needs Webpack. It is most beneficial for projects with high complexity and scale. For simpler projects, other tools or no bundler at all may suffice.