Enhancing Security with Prisma Middleware and Soft Delete
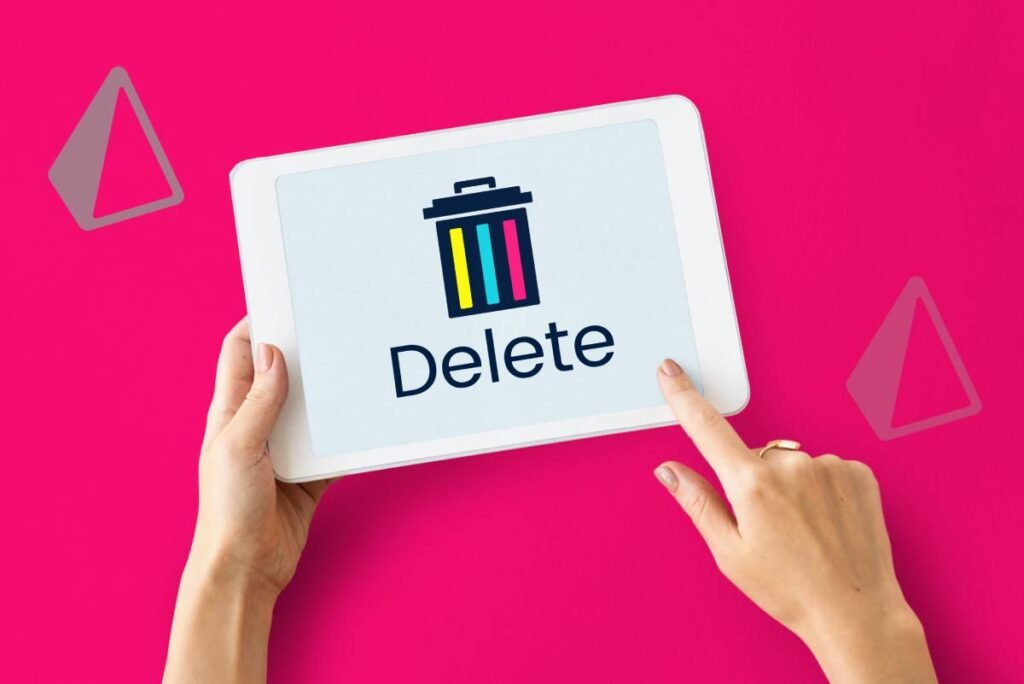
Data security is a top priority for modern applications handling sensitive user information. Prisma, a powerful ORM for Node.js and TypeScript, provides middleware capabilities that enable developers to enforce security policies seamlessly. One essential security measure is soft delete, which prevents permanent data loss while maintaining logical deletion states.
Table of Contents
Why Choose Soft Delete Over Hard Delete?
In traditional database operations, deleting a record means it’s gone for good—permanently erased. However, soft delete take a different approach by marking records as deleted without physically removing them. Instead of saying goodbye forever, you’re essentially tucking them away, making them invisible to normal queries but still accessible when needed.
- Instant Data Recovery – Mistakes happen. With soft deletes, a wrongly deleted record can be restored in seconds. No need for complex backups or database rollbacks.
- Stronger Security & Compliance – Many industries require data retention for audits, fraud detection, or legal reasons. Soft deletes ensure you’re compliant without cluttering the active dataset.
- Prevent Orphaned Data Issues – Deleting a parent record can leave child records dangling, causing referential integrity problems. Soft deletes keep relationships intact while hiding unwanted data.
- Protection Against Accidental Deletion – Instead of irreversible loss, soft deletes act like a safety net. If a critical record is mistakenly removed, it’s just a status update away from recovery.
However, implementing soft deletes requires ensuring that deleted records do not appear in normal queries. This is where Prisma middleware comes in.
Implementing Soft Delete with Prisma Middleware
Prisma middleware intercepts queries before they reach the database, making it an excellent tool for enforcing soft deletes.
1. Modify Your Prisma Schema
Add a deletedAt
column to mark deleted records:
model User {
id String @id @default(uuid())
name String
email String @unique
deletedAt DateTime? @default(null)
}
2. Automatically Exclude Soft-Deleted Records
Create a Prisma middleware function to filter out logically deleted records:
import { Prisma } from '@prisma/client';
export function softDeleteMiddleware(): Prisma.Middleware {
return async (params, next) => {
if (['findUnique', 'findFirst', 'findMany'].includes(params.action)) {
params.args.where = { ...params.args.where, deletedAt: null };
}
return next(params);
};
}
3. Intercept Delete Actions
Modify delete operations to update the deletedAt
field instead of permanently removing data:
export function interceptDeleteMiddleware(): Prisma.Middleware {
return async (params, next) => {
if (params.action === 'delete') {
params.action = 'update';
params.args.data = { deletedAt: new Date() };
} else if (params.action === 'deleteMany') {
params.action = 'updateMany';
params.args.data = { deletedAt: new Date() };
}
return next(params);
};
}
4. Register Middleware in Prisma Client
To apply the middleware, register it when initializing Prisma:
import { PrismaClient } from '@prisma/client';
import { softDeleteMiddleware, interceptDeleteMiddleware } from './middleware';
const prisma = new PrismaClient();
prisma.$use(softDeleteMiddleware());
prisma.$use(interceptDeleteMiddleware());
export default prisma;
When Not to Use Soft Deletes
While soft deletes are great for most scenarios, they may increase storage requirements and require manual cleanup for truly obsolete data. In cases where compliance requires complete removal (e.g., GDPR’s “right to be forgotten”), hard deletes might be necessary.
Best Practices for Secure Soft Delete
Soft deletes allow you to retain records without permanently erasing them, but improper implementation can lead to security risks, performance issues, and compliance violations. To ensure a reliable and secure approach, follow these best practices:
Automatically Exclude Soft-Deleted Records
By default, all queries should filter out soft-deleted records. This ensures that removed data remains hidden from standard operations unless explicitly retrieved. Implementing this at the database or application level prevents accidental exposure of deleted information.
Restrict Access Based on User Roles
Not every user should have access to deleted records. Use role-based permissions to determine who can view, restore, or permanently remove soft-deleted data. Limiting access reduces the risk of unauthorized recovery or data manipulation.
Set Up Automatic Data Cleanup
Retaining soft-deleted records indefinitely can lead to database bloat. Establish a scheduled process to permanently delete records after a specific period, balancing data retention needs with storage efficiency.
Maintain Logs for Accountability
Track every soft delete operation, including timestamps, user details, and reasons for deletion. Maintaining audit logs enhances transparency, helps detect anomalies, and ensures compliance with regulatory standards.
Ensure Consistency in Related Records
If a parent record is soft-deleted, all dependent records should also be marked as deleted. Implement cascading soft deletes to maintain referential integrity and prevent data inconsistencies.
Frequently Asked Questions (FAQs)
1. What happens if I try to query a soft-deleted record?
Prisma middleware will automatically exclude soft-deleted records unless explicitly queried.
2. Can soft deletes impact performance?
While soft deletes slightly increase storage usage, they improve safety and auditability. Optimized indexing mitigates performance issues.
3. How can I permanently delete records?
Set up a scheduled job to remove records where deletedAt
is older than a specific duration.
4. Can I apply soft deletes to multiple models?
Yes! Extend the middleware to include different models by modifying the params.model
condition.
5. Is it possible to restore soft-deleted records?
Yes, simply update deletedAt
back to null
to restore a deleted record.
Conclusion
Soft delete, when implemented with Prisma middleware, provides a secure and efficient way to manage data. By automating logical deletions, you safeguard against accidental data loss while ensuring compliance with regulatory requirements.
With the right middleware setup and best practices, you can enhance data security while maintaining application performance. For more on securing your applications, check out our guide on Fixing Node.js Security Vulnerabilities.