Getting Started with Fabric.js: A Guide for JavaScript Developers
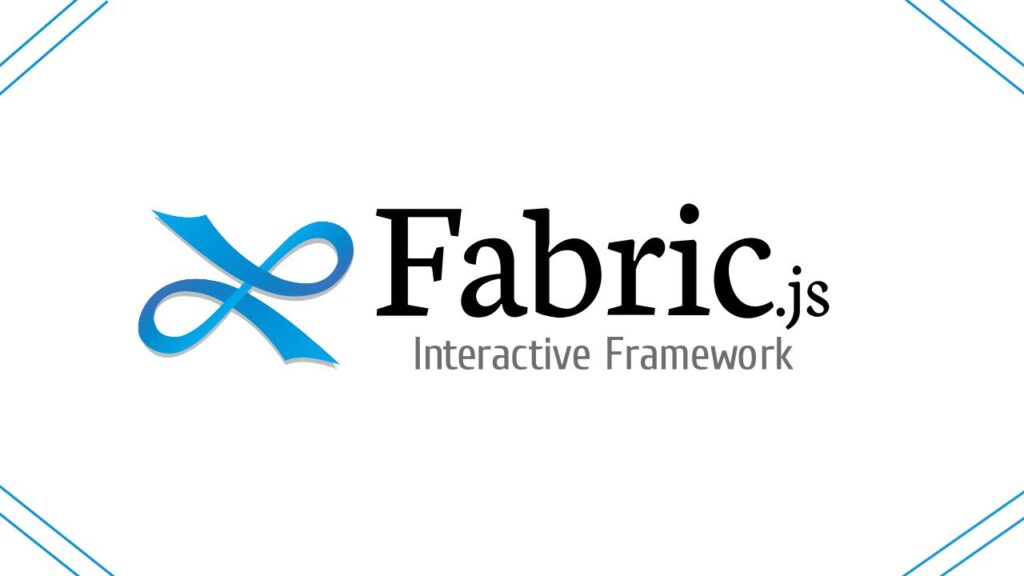
What is Fabric.js?
Fabric.js is a powerful and versatile JavaScript library that simplifies working with HTML5 Canvas. Designed for creating rich and interactive graphics, it provides an intuitive API that allows developers to manipulate images, shapes, and text easily. With over eight years of experience in JavaScript development, I can confidently say Fabric.js stands out as a powerful tool that enhances any web project.
Table of Contents
Key Features of Fabric.js
This library offers numerous features that make it easier to create engaging graphics. Among the most notable are its object model, which allows for easy manipulation of shapes and images, and its support for rich text functionality. Additionally, Fabric.js supports event handling and provides utilities for serialization, making it easier to save and restore canvas states. These features empower developers to create dynamic applications without the complexity often associated with graphic rendering.
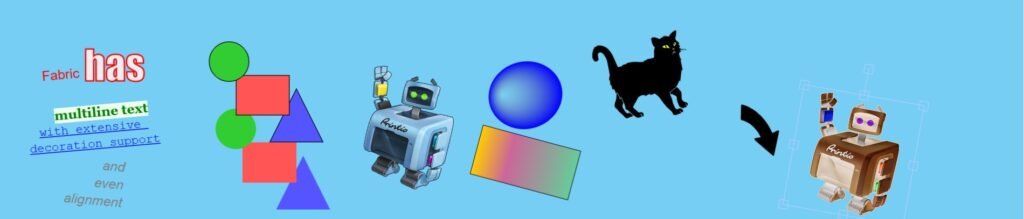
Why Use Fabric.js?
For JavaScript developers, learning Fabric.js can be incredibly beneficial. Its simplicity and flexibility reduce the learning curve associated with HTML5 Canvas, enabling you to implement complex graphics quickly. Furthermore, with a growing community and comprehensive documentation, developers can find ample resources and examples to get started. Whether you’re building an interactive web app or an online drawing tool, Fabric.js is an excellent choice that can elevate your project significantly.
- Simplifies Canvas API: The HTML5 Canvas API can be complex and verbose. Fabric.js abstracts much of this complexity.
- Rich Features: Offers advanced features like object grouping, event handling, and path manipulation.
- Cross-Browser Compatibility: Ensures your graphics work seamlessly across modern browsers.
- Extensible: Supports custom shapes, plugins, and extensions for specific needs.
Getting Started HTML Canvas tutorial with Fabric.js
To start using Fabric.js, you first need to include it in your project. You can do this using a CDN or npm.
Installation
Using a CDN
<script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/5.2.4/fabric.min.js"></script>
Using npm or yarn
npm install fabric --save
// or
yarn add fabric
Once installed, you’re ready to create your first Fabric.js canvas!
Core Features of Fabric.js
1. Creating a Canvas
The canvas is the foundation of all Fabric.js projects.
const canvas = new fabric.Canvas('c');
Add this to your HTML:
<canvas id="c" width="800" height="600"></canvas>
2. Drawing Shapes
Fabric.js makes it easy to add shapes like rectangles, circles, or polygons.
Example: Adding a Rectangle
const rect = new fabric.Rect({
left: 100,
top: 100,
fill: 'red',
width: 200,
height: 100,
});
canvas.add(rect);
3. Adding Images
Fabric.js simplifies image manipulation.
fabric.Image.fromURL('image-url.jpg', function (img) {
img.scale(0.5);
canvas.add(img);
});
4. Object Manipulation
Example: Rotating an Object
rect.rotate(45);
canvas.renderAll();
Real-World Use Cases of Fabric.js
- Graphic Design Tools
Tools like Canva or Figma rely on interactive canvas libraries for drag-and-drop design. - Image Editors
Fabric.js powers features like cropping, scaling, or adding overlays. - Whiteboards
Collaborative whiteboards use Fabric.js to allow drawing and interaction in real time. - E-Commerce Product Customization
Online stores use Fabric.js for customizing products like T-shirts, mugs, or posters.
Best Practices for Working with Fabric.js
- Optimize Performance: Use object caching and avoid unnecessary renders.
- Modularize Code: Keep your code organized by separating logic for different components.
- Event Handling: Use Fabric.js event listeners to create interactive experiences.
FAQ
Is Fabric.js free to use?
Yes, Fabric.js is open-source and free to use under the MIT license.
Can I use Fabric.js with React or Angular?
Absolutely! Fabric.js integrates seamlessly with modern frameworks like React, Angular, or Vue.
How does Fabric.js handle responsive designs?
Fabric.js provides methods to scale the canvas and its objects dynamically, making it suitable for responsive applications.
Are there alternatives to Fabric.js?
Yes, libraries like Konva.js and Paper.js are alternatives, but Fabric.js excels in simplicity and feature set for canvas graphics.
Conclusion
Fabric.js is a game-changer for developers looking to create dynamic, interactive graphics on the web. Its intuitive API, extensive feature set, and ease of use make it the perfect choice for projects ranging from simple illustrations to complex image editors.
Ready to explore the possibilities with Fabric.js? Start by setting up a basic canvas, experiment with shapes and images, and build something extraordinary!
Have questions or tips to share? Let us know in the comments below. Don’t forget to explore more JavaScript content on jsupskills.dev!